Window Z Order
I have seen quite a few requests for this functionality. It can be frustrating that swings implementation almost forces you to use a single window due to lack of "z order" control for multiple windows. In truth its not so much an MDI interface (since we can achieve that through the use of JInternalFrame), but more that we want to be able to have a window hierarchy to keep windows in a particular Z order.
Why?
Almost every single time I have seen this question asked, I have also seen people criticize the querier about it. I find it strange but I often see this happen when we do not know a solution. A very quick example would be you need a toolbar above your display. You obviously dont want your tools disappearing behind it when you click on the main window. You could accomplish this with JInternalFrame, however if you want to be able to drag the toolbar to say a different monitor that will not work. Now technically Java already provides this to a point, specifically with (J)Dialogs as children of a window. The problem arises when you need multiple layers of windows rather than just one.
A (not perfect) Solution
I have a simple library to accomplish this, however it does have limitations. Specifically there will be "flicker" sometimes when clicking on a different window. This is greatly reduced if you use undecorated windows; the flicker you are seeing is related to the activation of windows that is happening behind the scenes. This will also work for JavaFX since you can use a JFXPanel inside of the windows.
Download
How to use the library
Using the IJavaSwingMDIManager interface you can create your Z layers and child windows. It is recommended that you use the ZParent and ZChild windows provided in the library but you can provide your own windows if you desire. A quick overview of some of the methods:
Creating a manager:
There are several calls to create a new manager (createManager(...)). They all use some variation of these parameters:
- basewindow: You can provide the bottom most layer window or let the system create it for you
- z-layers: You can specify how many layers to create
- dialogCreator: You can specify your own methods to create the child and parent windows if you desire
Creating child windows
Automatically:
- createChildWindow: creates a window on the z level that you specify
Manually
- getZParent: Gets the parent window for a particular z level
- registerChildren: Register listeners on a child window
To manually create a child you will need to create the JDialog with the owner set as the parent of the Z level you want. You will then need to register that child with the service.
JDialog myDialog = new JDialog(mdiService.getZParent(0));
mdiService.registerChildren(myDialog);
I recommend you use the automatic method; you can specify the dialog creator if you just need to change the way the dialogs look or otherwise need access to the windows.
Creating a layered workspace:
public static void main(String[] args)
{
JFrame frame = new JFrame();
frame.setSize(new Dimension(800,800));
frame.setTitle("Base Window");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
IJavaSwingMDIManager mdiInterface = new WindowManager(frame,3);
JDialog dialog = mdiInterface.createChildWindow(0);
dialog.getContentPane().add(new JLabel("Child of Z 0"));
dialog.pack();
dialog.setVisible(true);
dialog = mdiInterface.createChildWindow(1);
dialog.getContentPane().add(new JLabel("Child of Z 1"));
dialog.pack();
dialog.setVisible(true);
dialog = mdiInterface.createChildWindow(1);
dialog.getContentPane().add(new JLabel("Child of Z 1"));
dialog.pack();
dialog.setVisible(true);
dialog = mdiInterface.createChildWindow(2);
dialog.getContentPane().add(new JLabel("Child of Z 2"));
dialog.pack();
dialog.setVisible(true);
}
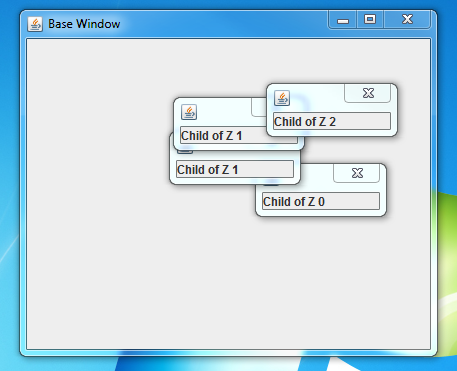
Children of a higher z order cannot go below children of a lower z order; children of the same z order can.
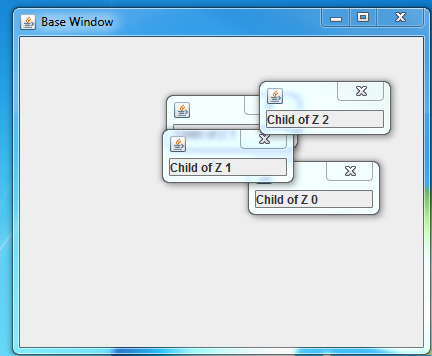
Enjoy!
Patricia Bradford