In a circle .....
A common request I see is for a layout that positions its children in a circle.
CircleLayout.java (5.5KB) accomplishes this by extending JavaFX's Pane layout. Here is a quick walkthrough of its highlights.
Important Properties
/**
* Stop Angle: The angle to layout the last child.
*/
public DoubleProperty stopAngleProperty();
public double getStopAngle();
public void setStopAngle(double value);
/**
* Start Angle: The angle to layout the first child. Default is 0 (top center)
*/
public DoubleProperty startAngleProperty();
public double getStartAngle();
public void setStartAngle(double value);
/**
* Inner radius: Layout will position the children in the outer ring
* of the circle. (Halfway between the inner radius and outer radius)
*/
public DoubleProperty innerRadiusProperty();
public double getInnerRadius();
public void setInnerRadius(double value);
/**
* Gets the outer radius of the circle. This is either the width or height
* whichever is smaller.
* @return radius
*/
public double getOuterRadius();
These allow you to layout the children in an arc around the circle starting and ending at any point along the circle.
For instance if i wanted to create a clock I might do something like this...
CircleLayout layout = new CircleLayout(100);
layout.getChildren().addAll(
new Label("12"),new Label("1"),new Label("2"),
new Label("3"),new Label("4"),new Label("5"),
new Label("6"),new Label("7"),new Label("8"),
new Label("9"),new Label("10"),new Label("11"));
Which looks like .....
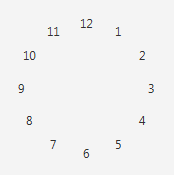
Or you could also produce the same results with this
CircleLayout layout = new CircleLayout(100);
layout.setStartAngle(30);
for(int i =1;i<13;i++)
{
layout.getChildren().add(new Label(i+""));
}
By default, If you do not set a stop angle, the layout will do a full circle. However it does support layout in a specific arc such as
CircleLayout layout = new CircleLayout(100);
layout.setStartAngle(30);
layout.setStopAngle(120);
for(int i =1;i<13;i++)
{
layout.getChildren().add(new Label(i+""));
}
Which produces this:
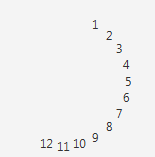
The layout also dynamically updates for certain changes such as:
Child size changes
Children added/removed
Layout size changes
Stop/Start angle changed
Inner radius changed
Enjoy!